ESlint & Prettier Configuration Link
1. You need to install these plugins
- ESLint
- Prettier – Code formatter
npm init @eslint/config or npx eslint –init
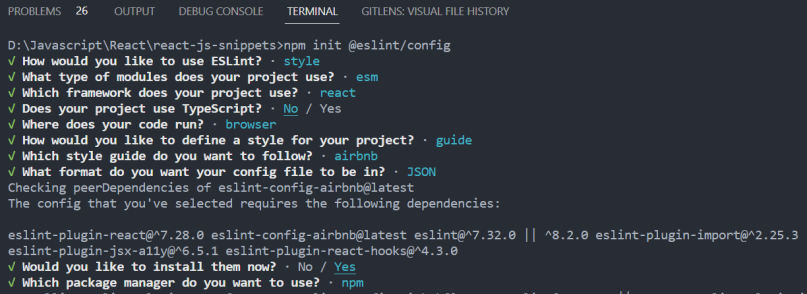
2. Or Manually Install Dev Dependencies
yarn add eslint-plugin-react eslint-config-airbnb@latest eslint --dev
yarn add prettier eslint-config-prettier eslint-plugin-prettier --dev
3. Create a .eslintrc.json file in your root folder
{
"env": {
"browser": true,
"es2021": true
},
"extends": [
"airbnb",
"eslint:recommended",
"plugin:react/recommended",
"plugin:@next/next/recommended",
"prettier"
],
"overrides": [],
"parserOptions": {
"ecmaVersion": "latest",
"sourceType": "module"
},
"plugins": ["react", "prettier"],
"rules": {
"react/jsx-no-bind": "off",
"jsx-a11y/click-events-have-key-events": "off",
"jsx-a11y/no-static-element-interactions": "off",
"arrow-body-style": "off",
"no-underscore-dangle": "off",
"no-unused-expressions": "off",
"jsx-a11y/anchor-is-valid": "off",
"jsx-a11y/anchor-has-content": "off",
"no-unused-vars": "off",
"react/function-component-definition": [
"error",
{
"namedComponents": ["function-declaration", "arrow-function"]
}
],
"react/jsx-filename-extension": [
1,
{
"extensions": [".js", ".jsx"]
}
],
"prettier/prettier": [
"error",
{
"trailingComma": "all",
"singleQuote": true,
"printWidth": 100,
"tabWidth": 4,
"semi": true,
"endOfLine": "auto",
"jsxSingleQuote": true,
"bracketSpacing": true
}
],
"react/react-in-jsx-scope": "off",
"react/prop-types": "off",
"react/jsx-props-no-spreading": "off"
}
}
4. In the settings.json file
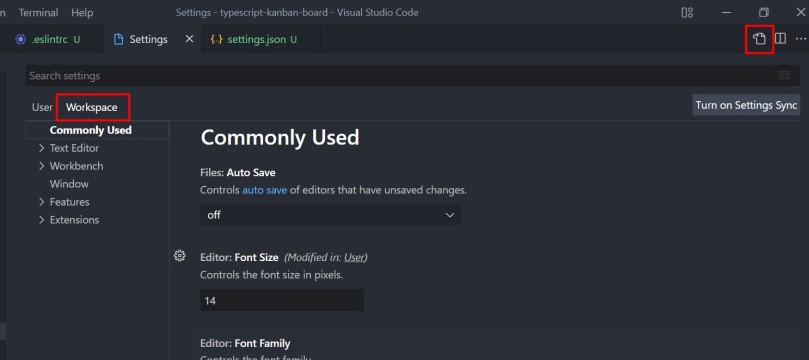
{
// config related to code formatting
"editor.defaultFormatter": "esbenp.prettier-vscode",
"editor.formatOnSave": true,
"[javascript]": {
"editor.formatOnSave": false,
"editor.defaultFormatter": null
},
"[javascriptreact]": {
"editor.formatOnSave": false,
"editor.defaultFormatter": null
},
"javascript.validate.enable": false,
"editor.codeActionsOnSave": {
"source.fixAll.eslint": true,
"source.fixAll.tslint": true,
"source.organizeImports": true
},
"eslint.alwaysShowStatus": true,
// emmet
"emmet.triggerExpansionOnTab": true,
"emmet.includeLanguages": {
"javascript": "javascriptreact"
}
}