1. Install Dev Dependencies
npm i -D eslint-config-standard-with-typescript@latest @typescript-eslint/eslint-plugin eslint eslint-plugin-import eslint-plugin-n eslint-plugin-promise typescript eslint-plugin-node eslint-config-node
npm i -D prettier eslint-plugin-prettier eslint-config-prettier
npm i -D nodemon jest supertest ts-jest ts-node @types/cors @types/express @types/jest @types/supertest
2. Create a .eslintrc.json file in your root folder
{
"env": {
"browser": true,
"es2021": true,
"node": true
},
"extends": [
"standard-with-typescript",
"plugin:node/recommended",
"plugin:prettier/recommended",
"prettier"
],
"overrides": [],
"parser": "@typescript-eslint/parser",
"parserOptions": {
"ecmaVersion": "latest",
"sourceType": "module",
"project": "./tsconfig.json"
},
"plugins": [
"@typescript-eslint",
"prettier"
],
"rules": {
"@typescript-eslint/no-var-requires": "off",
"@typescript-eslint/no-misused-promises": "off",
"@typescript-eslint/explicit-function-return-type": "off",
"@typescript-eslint/prefer-nullish-coalescing": "off",
"@typescript-eslint/strict-boolean-expressions": "off",
"node/no-unsupported-features/es-syntax": "off",
"node/no-unpublished-import": "off",
"node/no-missing-import": "off",
"node/no-missing-require": "off",
"arrow-body-style": "off",
"prefer-arrow-callback": "off",
"array-callback-return": "off"
}
}
Create .prettierrc.json
{
"arrowParens": "always",
"trailingComma": "es5",
"singleQuote": false,
"tabWidth": 2,
"printWidth": 100,
"semi": true,
"endOfLine": "auto"
}
.prettierignore to let Prettier know what files/folders not to format
build
3. In the settings.json file
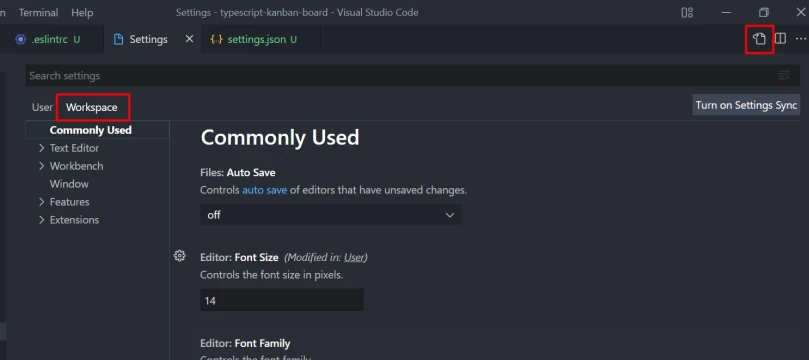
{
// config related to code formatting
"editor.defaultFormatter": "esbenp.prettier-vscode",
"editor.formatOnSave": true,
"[javascript]": {
"editor.formatOnSave": false,
"editor.defaultFormatter": null
},
"[javascriptreact]": {
"editor.formatOnSave": false,
"editor.defaultFormatter": null
},
"javascript.validate.enable": false,
"editor.codeActionsOnSave": {
"source.fixAll.eslint": true,
"source.fixAll.tslint": true,
"source.organizeImports": false
},
"eslint.alwaysShowStatus": true,
// emmet
"emmet.triggerExpansionOnTab": true,
"emmet.includeLanguages": {
"javascript": "javascriptreact"
}
}
4. Finally, we have to add the scripts in the package.json
"scripts": {
"start": "nodemon",
"build": "tsc",
"test": "jest",
"test:watch": "jest --watchAll",
"lint": "eslint src/**/*.{js,ts}",
"lint:fix": "eslint --fix src/**/*.{js,ts}",
"format": "prettier --check **/*.{js,ts}",
"format:fix": "prettier --write src/**/*.{js,ts}"
},